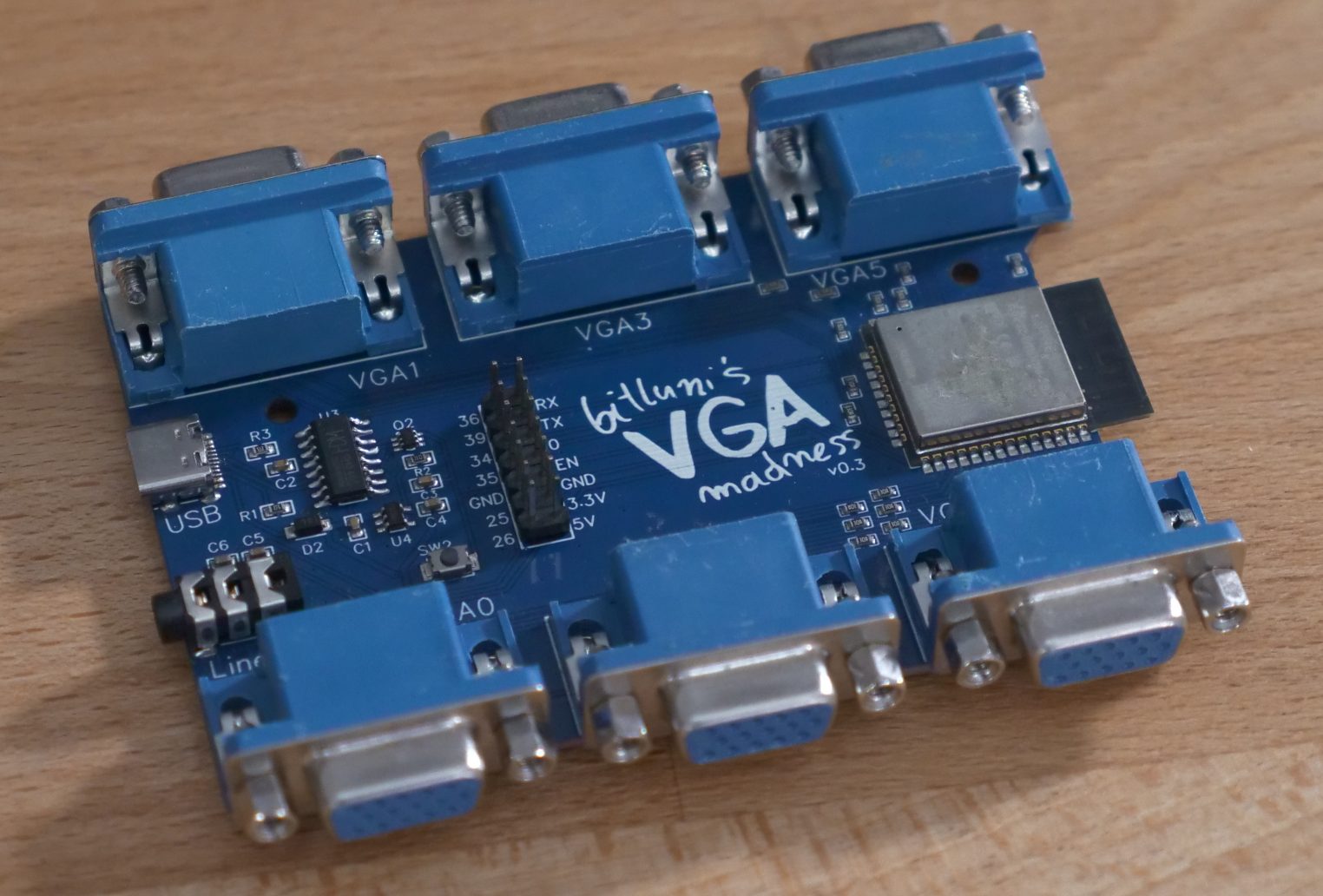
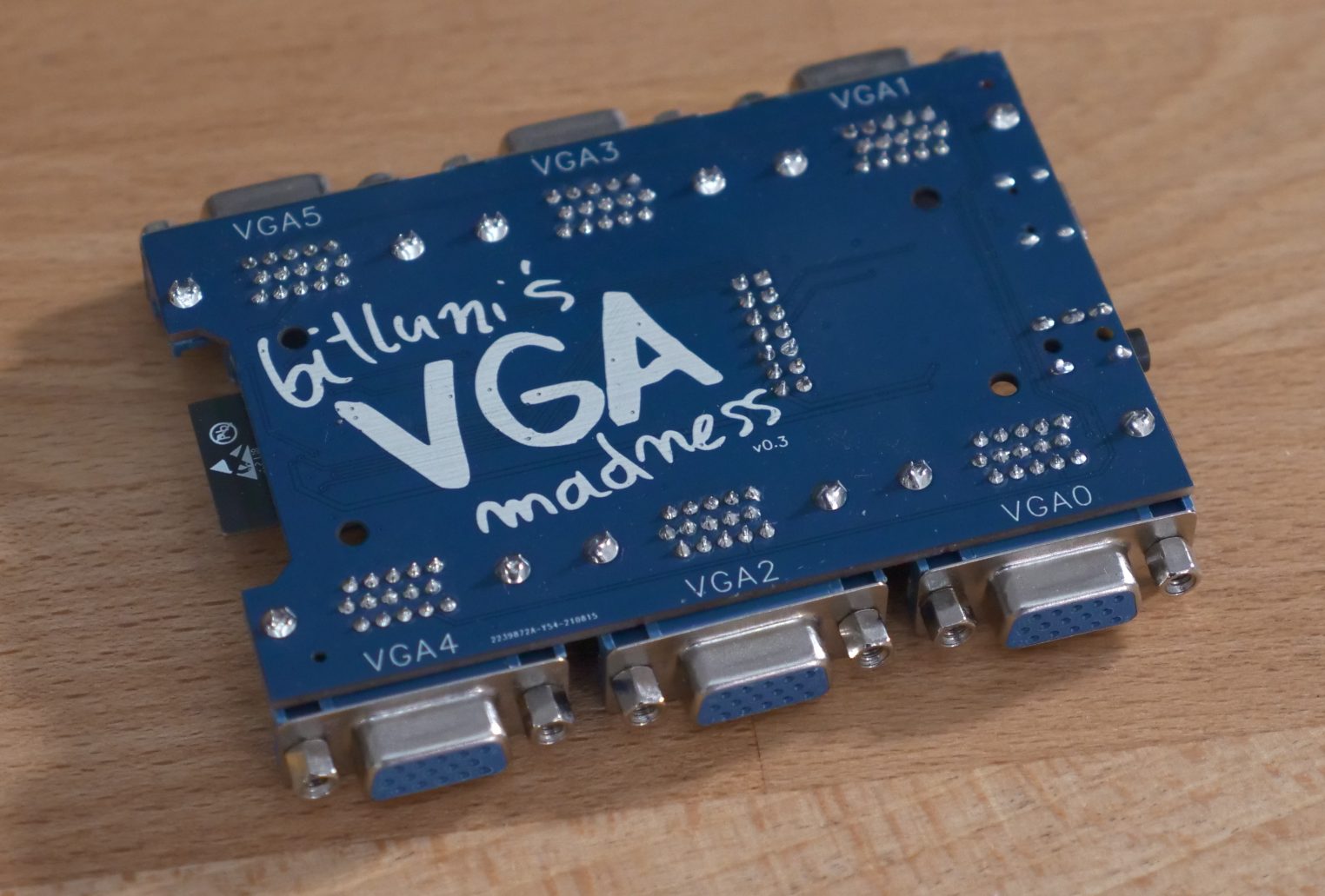
Hardware
VGAMadness is an ESP32 based board providing six VGA interfaces and a line out. The six interfaces share the sync signals so the same resolution is used simultaneously.
The pin configuration is as followed:
const int redPin0 = 17;
const int redPin1 = 2;
const int redPin2 = 19;
const int redPin3 = 12;
const int redPin4 = 23;
const int redPin5 = 26;
//green pins
const int greenPin0 = 16;
const int greenPin1 = 15;
const int greenPin2 = 18;
const int greenPin3 = 14;
const int greenPin4 = 22;
const int greenPin5 = 25;
//blue pins
const int bluePin0 = 4;
const int bluePin1 = 13;
const int bluePin2 = 5;
const int bluePin3 = 27;
const int bluePin4 = 21;
const int bluePin5 = 0;
const int hsyncPin = 32;
const int vsyncPin = 33;
The line out is utilizing the two internal DACs at pins 25/26. THere is no amplification but a 10uF capacitors in line for the AC coupling. It’s not enough to drive head phones but good enough as line out.
Software support
The board is supported by the Arduino library “bitluni’s ESP32Lib” v0.4 which can be found in the library manager of the Arduino IDE. This library provides all the functionality to drive all sorts of VGA boards. On top of that there is a lot of functionality to write text, draw and even display 3D graphics.
The VGA Madness board specifically with its pin configuration is provided with four examples showing different modalities.
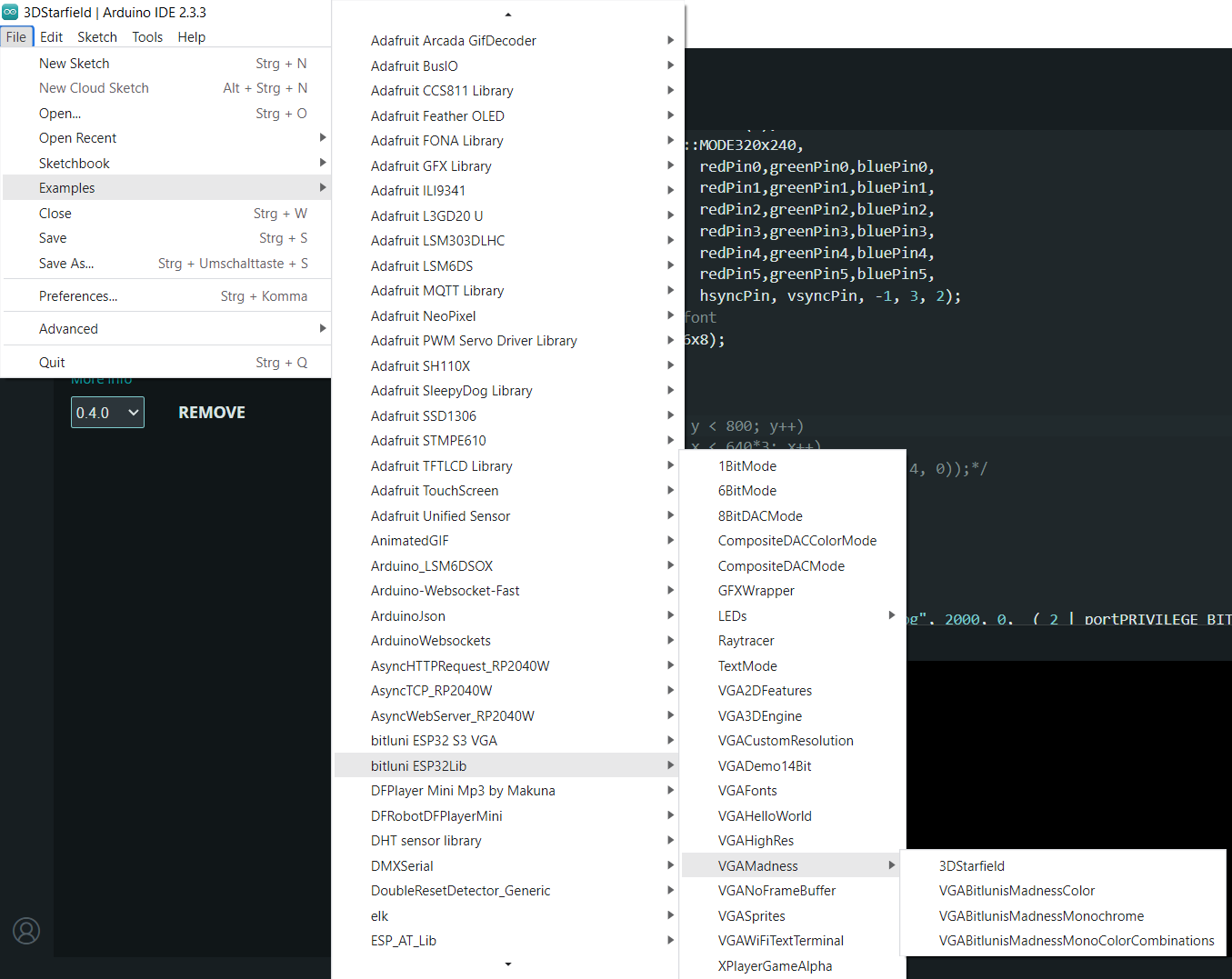
As target board “ESP32 Dev Module” needs to be selected. Please install esp32 by Espressif Systems v.3.0.x board support first.
Examples
3D Starfield is a basic example showing a monochrome starfield in a 3×2 screen configuration with double buffering. (Double buffering limits the resolution due to memory restrictions).
#include <ESP32Lib.h>
#include "VGA/VGA6MonochromeVGAMadnessMultimonitor.h"
VGA6MonochromeVGAMadnessMultimonitor gfx;
...
void setup()
{
gfx.setFrameBufferCount(2);
gfx.init(VGAMode::MODE320x240,
redPin0,greenPin0,bluePin0,
redPin1,greenPin1,bluePin1,
redPin2,greenPin2,bluePin2,
redPin3,greenPin3,bluePin3,
redPin4,greenPin4,bluePin4,
redPin5,greenPin5,bluePin5,
hsyncPin, vsyncPin, -1, 3, 2);
The two line from the setup configure the double buffer (single buffer is default) and initialize the VGA.
In the loop
gfx.clear();
...
gfx.show();
clear the backbuffer and swap the front and backbuffer at the end after the frame is redrawn. Using double buffering prevents flickering due to slow drawing and overlapping graphics. If the frame is redrawn in one go double buffering can be avoided.
This example is shown here:
VGAbitlunisMadnessColor is an example using 4 Screen with 3Bit Color at a time. To achieve this the I2S needs to run in 16Bit mode limiting the bandwidth and resolution. This example doesn’t use double buffering but shows simple static shapes and numbering of the screens.
VGAbitlunisMadnessMonochrome is a basic example displaying the numbers of the screens in 1Bit
VGAbitlunisMadnessMonoColorCombinations utilized the fact that the pixel signal doesn’t need to be sent to all 3 color components. The gpio matrix allows to route static 0 and 1 to any GPIO. This allows to use different 3bit colors per screen instead of black/white. Highly experimental.
Too get familiar with different functions the graphics interface provides please check out the other examples.